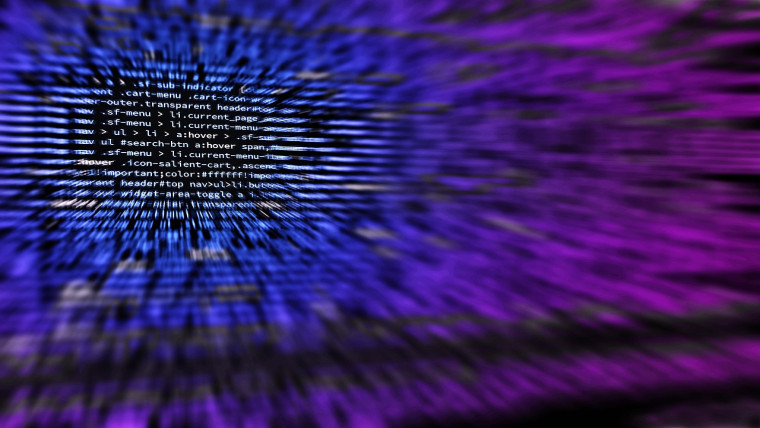
Laravel, a leading PHP framework, is celebrated for its elegant syntax, comprehensive toolkit, and a thriving ecosystem that empowers developers to build robust and scalable web applications. From Livewire to Inertia.js and Nova, the Laravel ecosystem is rich with tools that simplify and enhance development. This article explores these tools, delves into how Vue and React can be integrated using Inertia, explains the MVC (Model-View-Controller) architecture and Eloquent ORM, and discusses the benefits of Laravel for SaaS projects, particularly with Laravel Spark.
Understanding MVC in Laravel
The MVC (Model-View-Controller) architecture is foundational to Laravel, promoting organized and maintainable code. Each component has distinct responsibilities:
Model: Handles the business logic and data interactions. In Laravel, Eloquent ORM provides a powerful and expressive syntax for interacting with the database.
View: Responsible for the user interface and presentation of data. Blade templating engine simplifies the creation of dynamic and reusable views.
Controller: Acts as an intermediary, handling user input, processing it via the model, and returning the appropriate view.
This separation of concerns ensures that each part of the application is focused on a single responsibility, making the application easier to manage and scale.
Eloquent ORM
Eloquent ORM is Laravel's built-in Object-Relational Mapping tool, which allows developers to interact with the database using PHP syntax. It abstracts the complexity of SQL queries, providing an elegant and readable syntax.
Defining Models: Each database table has a corresponding "Model" in Laravel. For instance, a User model corresponds to a users table in the database.
class User extends Model
{
protected $fillable = ['name', 'email', 'password'];
}
Relationships: Eloquent makes it easy to define relationships between models, such as one-to-many, many-to-many, and one-to-one.
class Post extends Model
{
public function user()
{
return $this->belongsTo(User::class);
}
}
The Laravel Ecosystem
Laravel's ecosystem includes a variety of tools that enhance its core functionalities:
- Livewire
- Inertia.js
- Nova
- Laravel Spark
Livewire
Livewire is a full-stack framework for Laravel that allows developers to build dynamic interfaces without leaving the comfort of Laravel. It provides a way to create reactive components using PHP instead of JavaScript.
Real-time Updates: Livewire components can be updated in real-time as users interact with them.
Server-side Rendering: Since Livewire handles reactivity on the server, it improves SEO and performance by rendering fully on the server.
Inertia.js
Inertia.js offers a modern approach to building single-page applications (SPAs) without the need for a separate API. It allows the integration of Vue.js and React directly into Laravel applications.
Using Vue.js: Inertia.js makes it straightforward to use Vue.js within Laravel. Components are created as Vue components, enabling a reactive and interactive user experience.
Using React: Similarly, React can be used to build components. Inertia.js handles the communication between Laravel's backend and React frontend seamlessly.
Seamless Navigation: Inertia.js enables SPA-like navigation, where page transitions happen without full page reloads, enhancing the user experience.
Nova
Nova is a beautifully designed administration panel for Laravel applications. It provides an intuitive interface for managing application data and resources.
User-friendly Interface: Nova offers a sleek, user-friendly interface for administrators.
Customizable: Developers can extend Nova with custom tools, cards, and metrics to fit specific needs.
Resource Management: Easily manage Eloquent models and relationships directly from the Nova dashboard.
Laravel Spark
Laravel Spark is a SaaS (Software as a Service) starter kit that provides boilerplate for subscription-based applications. It includes features like user authentication, subscription billing, team management, and invoicing out of the box.
Subscription Management: Spark integrates with Stripe and Paddle for managing subscriptions and billing.
Authentication and Authorization: Provides robust authentication mechanisms and role-based access control.
Team Management: Supports creating and managing teams, assigning roles, and handling team-specific billing.
Benefits of Laravel for SaaS Projects
Laravel, combined with tools like Spark, offers numerous advantages for building SaaS applications:
Rapid Development: Laravel's expressive syntax and built-in features speed up development. Tools like Laravel Mix streamline asset compilation.
Scalability: Laravel is designed to scale. Features such as job queues, task scheduling, and caching ensure that applications can handle high traffic and large datasets.
Security: Laravel provides built-in security features, including CSRF protection, encryption, and secure password hashing. Comprehensive Ecosystem: The Laravel ecosystem, including Livewire, Inertia.js, and Nova, provides all the tools needed to build modern web applications efficiently.
Community Support: Laravel has a large and active community, extensive documentation, and numerous tutorials, making it easier to find help and resources.
Conclusion
The Laravel ecosystem is a powerhouse for modern web development, offering tools and frameworks that simplify and enhance the development process. From the dynamic interfaces enabled by Livewire to the seamless SPA capabilities of Inertia.js and the powerful administration panel provided by Nova, Laravel equips developers with everything they need to build robust applications. Coupled with the MVC architecture and Eloquent ORM, Laravel stands out as an excellent choice for developing SaaS projects, particularly with the help of Laravel Spark. Its rapid development capabilities, scalability, security features, and supportive community make Laravel an ideal framework for building sophisticated, high-quality web applications.