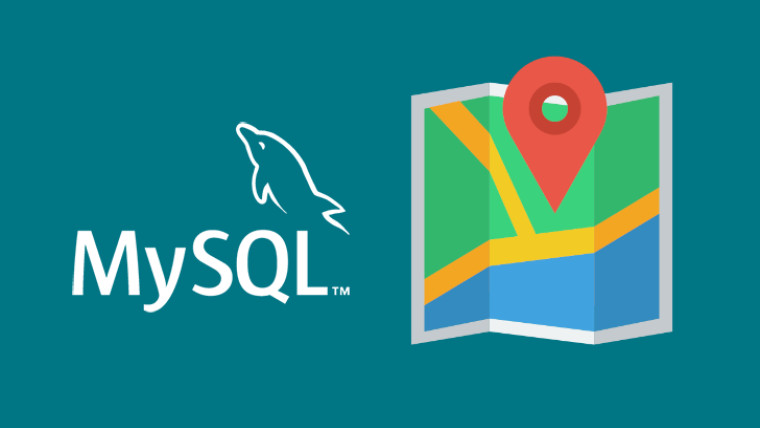
It's pretty easy to seed a geospatial point to a Laravel DB once you sort through the cruft of people telling you to do it this way or another. You need to use DB::RAW for a start and then ensure that your SQL for DB:RAW is in the format that MYSQL is expecting.
I won't waffle on just to get search / seo hits. This is what you need if you are using MySQL 5...
DB::raw("(GEOMFROMTEXT('POINT(54.8765696,-2.9261824)'))")
...or the following if using MySQL 8...
DB::raw("(ST_GeomFromText('POINT(54.8765696 -2.9261824)'))")
This is would insert a point on-top of Carlisle station.
In a seeding array this might look something like...
DB::table('places')->insert([['id' => 12, 'location' => DB::raw("(GEOMFROMTEXT('POINT(54.8765696,-2.9261824)'))")]]);
or
DB::table('places')->insert([['id' => 12, 'location' => DB::raw("(ST_GeomFromText('POINT(54.8765696 -2.9261824)'))")]]);
Obviously you'd need a reference to the DB facade for this to work but you've probably already got that....
use Illuminate\Support\Facades\DB;
This answer was trawled up from https://stackoverflow.com/questions/28645394/how-to-insert-spatial-point-values-with-a-query-in-mysql-in-laravel/32619541